Informal Science Education II
Detailed Design
EEL 5881 - Software Engineering - Fall 2000
Modification history:
Version | Date | Who | Comment |
Version 0.0 | August 15,
2000 | G. H. Walton | Template |
Version 1.0 | November
20, 2000 | Michael Wales | Initial document |
Vesrsion 2.0 | December 6, 2000 | Jeffrey Miller | Update |
Team Name:Miles Computer Engineering
Team Members:
Contents of this Document
Design Issues
Detailed Design Information
Trace of Requirements to Design
Design Issues:
- Maintainability: We really want to focus on creating this
product in a way that will provide for easy maintenance. We want to
follow good software engineering practices like data hiding, good
cohesion, and low coupling. Our lack of experience in software
engineering is making this a learning experience. The GUI components
in Java don't tend to make it easy for us to preserve our good software
engineering practices. Our worries were relieved when we consulted
Dr. Franceschini and he told us that GUI's typically don't follow good
code practices, it's their nature. We will still strive to create a
product that will be easily maintained.
- Java: Java was chosen so that we could make a graphical
program that was platform independent. Using Java, it looks like this
goal will be easily achieved. Java has proven to be very portable, and
an overall very powerful language to program in. As our experience
with the language grows, we become more appreciative of many of the
features of Java, and dissapointed at some omissions. One thing I have
learned that I will remember in future projects is Java's performance.
Java's performance on a 486 is really poor, for the relatively
simple program we have created. A 486 could easily handle many of these
programs running at the same time in it's native language. If this
program had critical timing, we probably wouldn't be able to use Java on
anything less than a Pentium II.
- Inexperience with GUI programming: This has been the hardest
aspect of the program by far. Our old programming practices simply
don't work the same in these event controlled environments. To use the
GUI components, we have had to add a lot of different code and classes
that we really didn't expect to need when we first started. If a
software engineer ever has to maintain our product at a later date and
senses that we went the wrong way on our design, this is probably why.
Our design from when we started and where we will finish will be
dramatically different because of this too.
Detailed Design Information:
UML Static Structure Diagrams
TransitionWindow and the classes around it: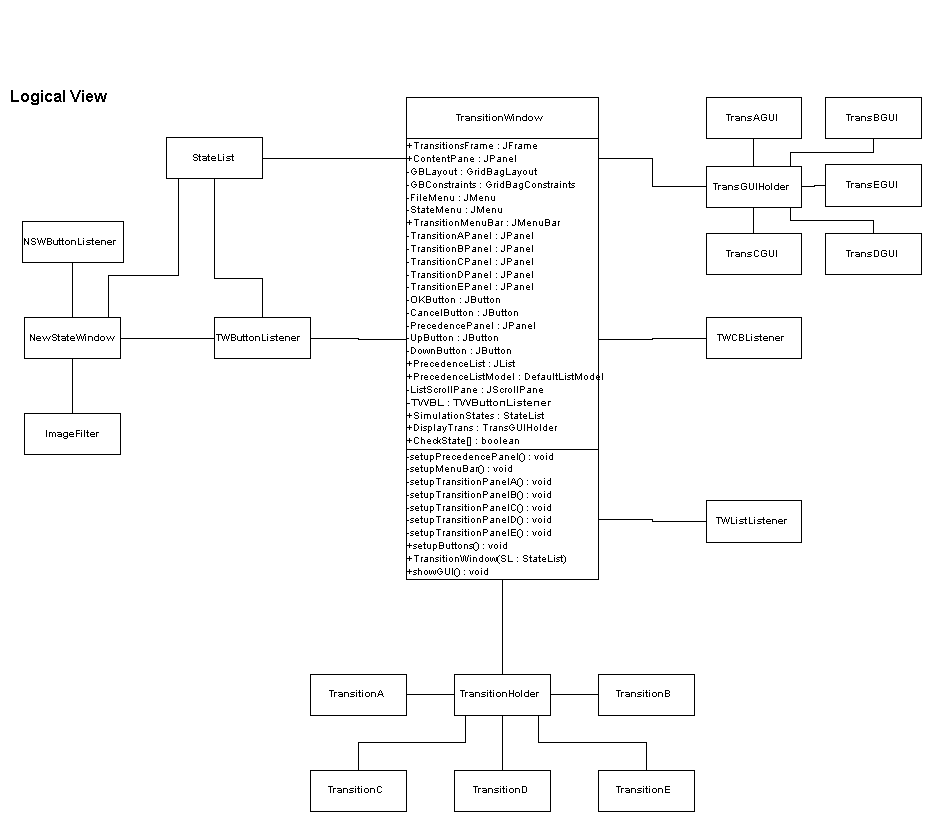
StateList and the classes around it: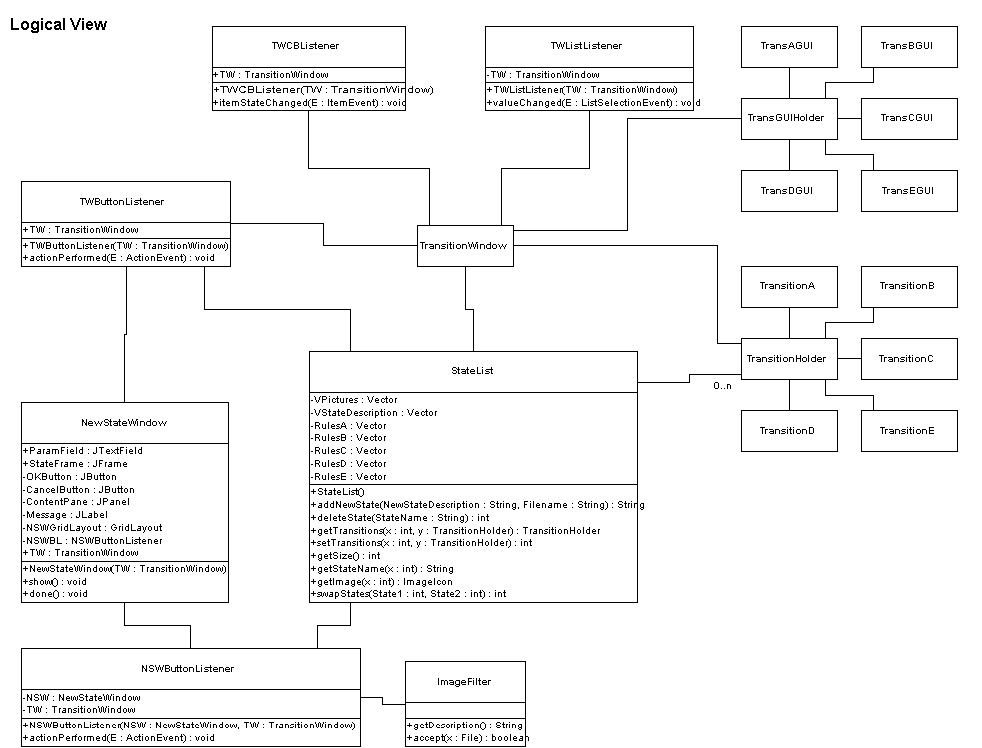
TransGUIHolder and the classes around it: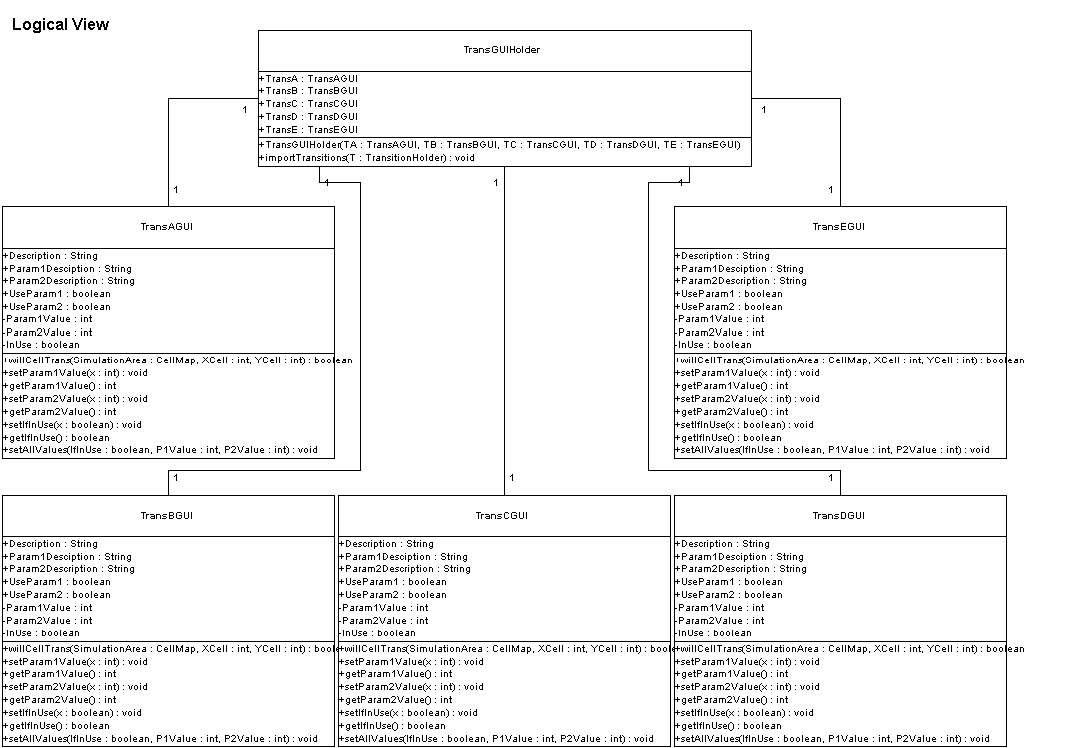
TransitionHolder and the classes around it: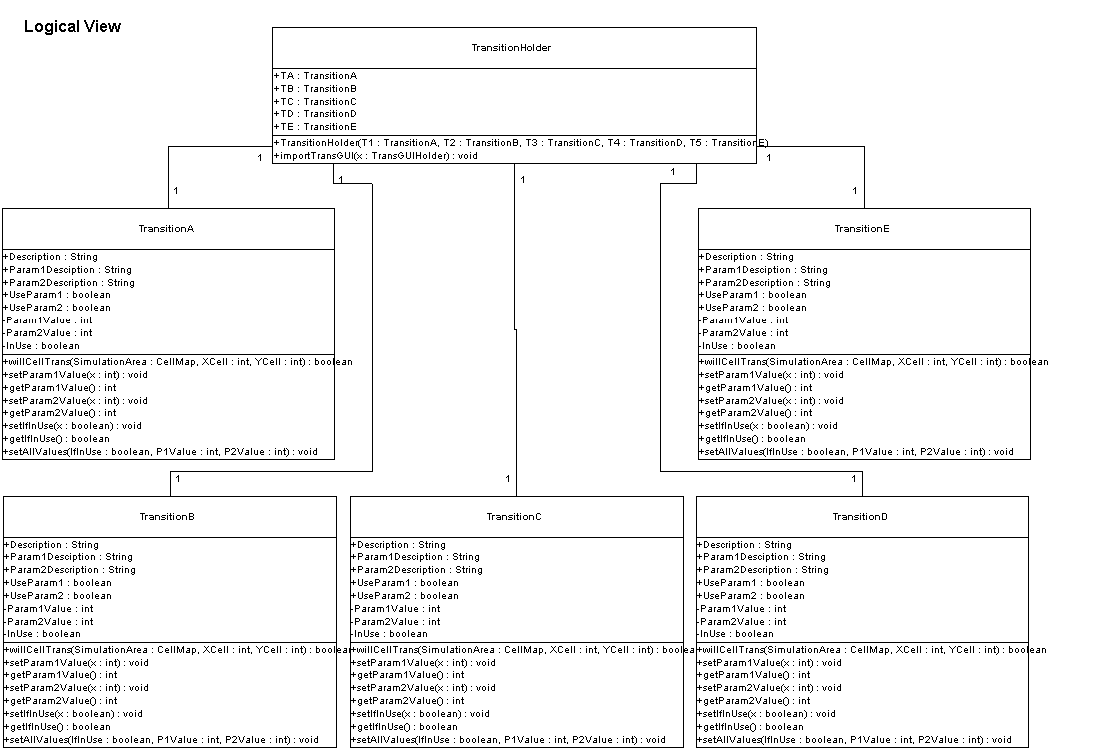
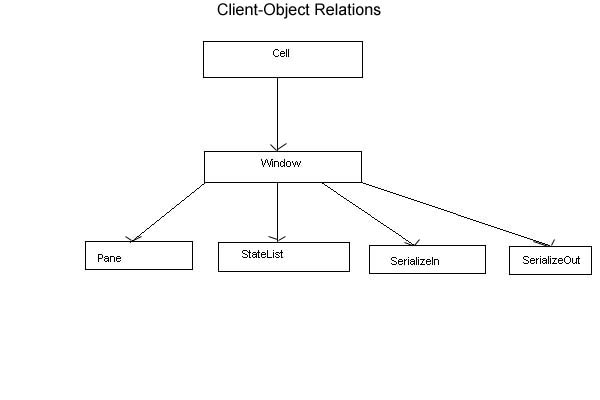
Descriptions of Classes:
- ImageFilter: This class is used to filter image files when
using a JFileChooser dialog box. Neither of these methods will
be explicitly called by any code in the program, they will handled by
the JFileChooser class. The filter will only show directories,
gif files, jpeg files, and tiff files.
- String getDescription(): Returns the description of the
filter so it can be displayed on the filter list.
- boolean accept(File): Returns true if the file passed
should be displayed or not. This simply verifys that the file
has the correct extension on it's filename.
- NewStateWindow: This class is instantiated when the user
selects that they wish to create a new state from the transition and
state editting menu bar. This class has to create a new popup
JFrame for the user to input the name of the state. After the user
inputs the name of the state, the JFileChooser pops up and asks
the user to select the image file (with the help of the ImageFilter
). When the user selects a image, all the data is sent to the
StateList class, and if it is accepted the GUI is updated to
reflect the changes. If the StateList class rejects the state,
the reason for the error is displayed to the user. The
NewStateWindow has it's own listener for buttons.
- void done(): This method is called after the new state
is created to clean up the variables. It dereferences and
makes the windows no longer in use invisible.
- Constructor NewStateWindow(TransitonWindow): The
constructor is passed the TransitionWindow class so it
knows what object to update with the new state. The constructor
also initializes all the GUI components of it's JFrame.
- void show(): This method is called to show the frame for
new state window. Called directly after the constructor.
- NSWButtonListener: This class handles all the button clicks for
the NextStateWindow class. This class is also responsible for
verifying that the user's inputs were accepted by the StateList
class.
- void actionPerformed(ActionEvent): This method is called
each time the user clicks a button on the NextStateWindow
class' GUI. If the user clicks cancel, the window closes. If
the user selects OK, it opens the JFileChooser so that
the user will pick the correct file for the state that they are
creating.
- Constructor NSWButtonListener(NextStateWindow, TransitionWindow): The
constructor is passed the NextStateWindow and the
TransitionWindow that it will be modifying the contents
of.
- StateList: This class holds all the information on the
simulation's current states and the current parameters for the
transition rules. Each state has a description string and an image that
will be displayed on the main simulation screen. All the descriptions
are stored in a vector VStateDescription. Each transition rule
is stored in vector also. The methods below provide the interface to
the rest of the program and make sure the parameters passed to the
methods are within the correct limits. The order of the states is the
order of precedence that the states will transition into. If a cell
can transition into both state #3 and state #5, it will transition into
state #3 because it is higher on the StateList.
- String addNewState(String Description, String Filename):
This method loads the image at the specified at the filename
given and verifies that the image is a 20x20 image. If it is not
the correct size or any type of error occurs, the method returns
back an error message. If everything goes well, and the new
state is added correctly, the method will return a null string.
- int deleteState(String): The method will find the state
named in the string, and remove it. It returns 0 on a successful
deletion.
- ImageIcon getImage(int): Returns the ImageIcon
corresponding to the state number passed to the method. It will
return a null image if the state number was invalid.
- int getSize(): Returns the number of states in the
StateList.
- String getStateName(int): Returns the string description
of the state corresponding to the integer passed to the method.
- TransitionHolder getTransitions(int, TransitionHolder): This
method is passed the number corresponding to the state that the
program needs the transitions for, and the TranstionHolder
object where it should store the transitions. It also returns the
same TransitionHolder. The TransitionHolder will be
filled with all the transition objects that the caller requested.
- int setTransitions(int, TranstionHolder): This method is
passed the state number and set of transitions to use for that
state. If the operation is succesful the method will return 0.
- Constructor StateList: Initializes the vector classes.
- int swapStates(int, int): This method will swap the
order of the two state's numbers that are passed to the method.
If either of the state numbers are invalid, nothing will happen
and the return a non-zero number.
- TransAGUI, TransBGUI, TransCGUI, TransDGUI, TransEGUI: These
classes are the corresponding GUI components for the TransitionA,
TransitionB, TransitionC, TransitionD, and TransitionE
classes. Each class sets up a unique transition property, but each
class has each of the following methods. The variables name give the
obvious use of each variable. The static variables describe the
actual transition to the user. The variables Param1Value,
Param2Value, and InUse will differ for each state. InUse
is the variable representing the status of the checkbox.
- boolean getIfInUse(): Returns true if the transition is
currently set to be used.
- int getParam1Value(), int getParam2Value(): These
methods will return the value of the first and second parameters
respectivly. These will not neccesarilly be the current values
in the JTextField.
- void setAllValues( boolean, P1 int, P2 int): Sets all
three variables of the current transition. The first parameter
sets the IfInUse variable, and the second two set the
Param1Value and Param2Value variables.
- void setIfInUse(boolean): If passed true, sets the
transition rule to be used when determining a cell transition for
the corresponding state.
- void setParam1Value(int), void setParam2Value(int):
These methods are used to set the values for Param1Value
and Param2Value.
- TransGUIHolder: This class is instantiated by the
TransitionWindow class. The class contains the parameters and
the components used by the properties window. It exists in the program
in 2 forms. Form 1 is a single object representing what is on the
screen currently. Form 2 is an array, 1 index for each state, that
represents the temporary parameters for each state. If the user hits
the OK button, all these parameters are stored into the Transition
classes, if the cancel button is clicked, then all the data is thrown
away. The class simply contains the five different transition
GUI components (TransAGUI, TransBGUI, TransCGUI, TransDGUI,
and TransEGUI).
- Constructor TransGUIHolder( TransAGUI, TransAGUI, TransBGUI,
TransCGUI, TransDGUI, TransEGUI): Constructor simply
provides a quick way to assign each of the attributes an object.
- TransitionWindow: This class is instantiated when the main
simulation calls for it after the user selects that they need to alter
the state and transition properties from the menu bar. Most of the
attributes of this class are used internally by the listeners of
TransitionWindow and are simply the GUI objects.
SimulationStates is the StateList that is passed to the
constructor from the main simulation class. DisplayTrans is the
class that is actually displayed on the properties window. There is
also an array of TransGUIHolder to temporarily hold all the
user's selections before they hit the OK button. CheckState is
an array of the current values of all the checkboxes.
- void setupPrecedencePanel(), void setupMenuBar(),
void setupTransitionPanelA(), void setupTransitionPanelB(),
void setupTransitionPanelC(), void setupTransitionPanelD(),
void setupTransitionPanelE(), and void setupButtons(): These
methods are all internal to the class, and should not be called by
anything outside of this class. As the names of the methods
indicate, they are all used in setting up the GUI.
- Constructor TransitionWindow( StateList ): Main
constructor for the program. The StateList passed is the one
that the properties window will be used to edit. This class
instantiates all the GUI components and gets them ready for
display.
- void showGUI(): Called when the main simulation class wants
the property window displayed. Should immediantly follow the
constructor TransitionWindow( StateList ).
- TransGUIHolder: This class is instantiated by the
TransitionWindow class. The class contains the parameters and
the components used by the properties window. It exists in the program
in 2 forms. Form 1 is a single object representing what is on the
screen currently. Form 2 is an array, 1 index for each state, that
represents the temporary parameters for each state. If the user hits
the OK button, all these parameters are stored into the Transition
classes, if the cancel button is clicked, then all the data is thrown
away. The class simply contains the five different transition
GUI components (TransAGUI, TransBGUI, TransCGUI, TransDGUI,
and TransEGUI).
- Constructor TransGUIHolder( TransAGUI, TransAGUI, TransBGUI,
TransCGUI, TransDGUI, TransEGUI): Constructor simply
provides a quick way to assign each of the attributes an object.
- void importTransitions(TransitionHolder): This will save
the data stored in the TransitonHolder into the
TransGUIHolder object.
- TransitionA, TransitionB, TransitionC, TransitionD, TransitionE:
These classes store the information used to determine how each state
and cell will transition after each clock period during the simulation.
These classes are very similar to their corresponding TransGUI
classes. These classes should be altered if the user would like to
create new sets of transition rules not provided in the program.
- boolean getIfInUse(): Returns true if the transition is
currently set to be used.
- int getParam1Value(), int getParam2Value(): These
methods will return the value of the first and second parameters
respectivly. These will not neccesarilly be the current values
in the JTextField.
- boolean willCellTrans(CellMap, int x, int y): This
method is the key method used in the simulation. The simulation
will pass the map object to the method, and the x and y
coordinates of the cell in question. If the cell will
transition to this state (the state that this transition is
associated with) the method returns true. This method will be
the key method that will be reprogrammed by a user who wishes to
develop his own transition rules.
- void setAllValues( boolean, P1 int, P2 int): Sets all
three variables of the current transition. The first parameter
sets the IfInUse variable, and the second two set the
Param1Value and Param2Value variables.
- void setIfInUse(boolean): If passed true, sets the
transition rule to be used when determining a cell transition for
the corresponding state.
- void setParam1Value(int), void setParam2Value(int):
These methods are used to set the values for Param1Value
and Param2Value.
- TransitionHolder: This class is used to send the Transition
objects between classes. It also provides a method to easily
convert a TransGUIHolder class into a TransitionHolder
class. This will be used when the data that is stored in the
temporary TransGUIHolder objects from the transition editting
window is accepted and will be loaded into TransitionA, TransitionB,
TransitionC, TransitionD, and TransitionE.
- Constructor TransitionHolder(TransitionA, TransitionB,
TransitionC, TransitionD, TransitonE): This provides a
quick and easy way to load the holder up with objects.
- void importTransGUI(TransGUIHolder): This will save
the data stored in the TransGUIHolder into the
TransitionHolder.
- TWCBListener: This class is used to keep track of the status
of each checkbox. Unfortunately in Java, there is no method that will
return the state of a JCheckBox. This method updates the
CheckState[] variable in the TransitionWindow class.
- void itemStateChanged(ItemEvent): This method is
executed everytime one of the checkboxes are clicked. It
determines the new state of the checkbox, and saves it to the
CheckState[] variable in the TransitionWindow class.
- Constructor TWCBListener(TransitionWindow): The
constructor is passed the TransitionWindow class so that
the class can figure out which checkbox was being clicked, and
change the status of the CheckState[] variable.
- TWButtonListener: This is the TransitionWindow's most
complex listener because it handles so many different events. All the
menu bar commands and all the buttons on the GUI are handled by the
listener.
- void actionPerformed(ActionEvent): This method is
executed each time a menu bar item is selected or a button is
clicked. The method first gets the message sent by the object
that called the method. Each GUI component will send a unique
message identifying itself, so that the listener can handle the
action accordingly.
- Constructor TWButtonListener(TransitionWindow): This
constructor is simply passed the TransitionWindow class
so that the class knows where all the events are coming from.
- TWListListener: This class is the listener for the
TransitionWindow's list. When the list is clicked, whatever item
is selected will be have it's parameters displayed on the right hand
side of the screen. The parameters that were being displayed will be
stored temporarily until the user decides to accept or throw away the
changes by hitting the OK button.
- Constructor TWListListener(TransitionWindow): The
constructor is passed the TransitionWindow class so that
the GUI components stored in TransitionWindow can be
modified.
- void valueChanged(ListSelectionEvent): This method is
executed when the user clicks on the list. This method stores
the current contents of the GUI components on the right hand side
of the window, and loads the parameters for the selected state.
- cell: This class provides the executable file for the user.
- Constructor: none
- public static void main(String args[]): This method
provides the starting point of the program.
- window: This is class for the main simulation window.
- Constructor: Creates the frame and the menu bars which will
be displayed. Also sets up a blank pane to begin.
Final Class Structure
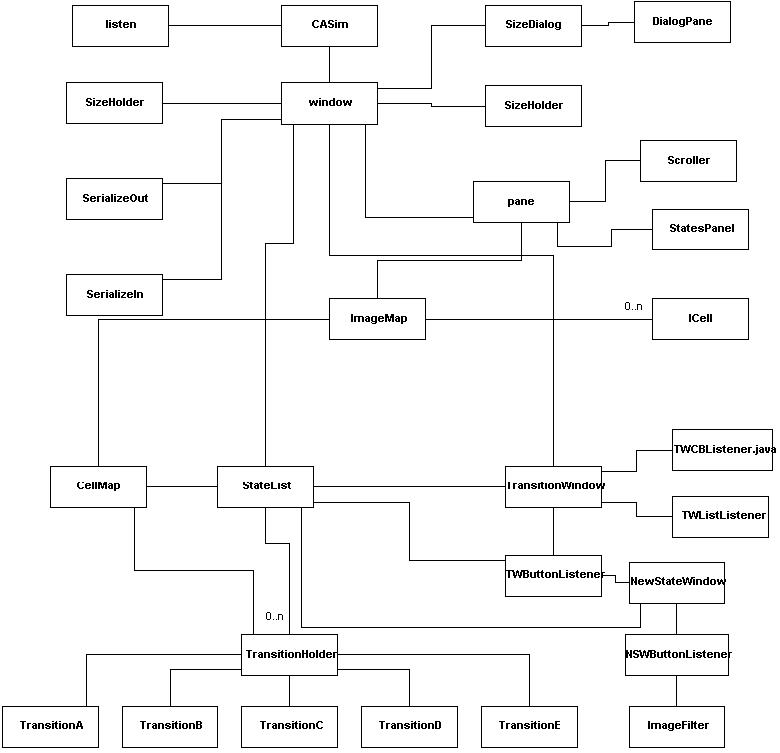
Trace of Requirements to Design:
Req. No. | Location of implementation |
No. 1 | cell class will provide a user friendly
editting environment for the user to change the initial conditions of
the simulation. |
No. 2 | The TransitionWindow class will provide the
user an interface to change the transition parameters for each state.
The user can reprogram the transition rules by recoding the following
transition classes and the transition's GUI classes. |
No. 3 | The user will edit the way the simulation will be
executed through interfaces provided in the cell class. |
No. 4 | The simulation will be saved through the cell
class using one of the serialization classes. The transition and state
properties will be saved through the TransitionWindow's GUI
interface. |
No. 5 | The TransitionA and TransGUIA (and
similar classes) will provide a way for the user to easily reprogram
the transition rules. |
No. 6 | This functionality will be provided by the
TransitionWindow and NewStateWindow classes. |
No. 7 | This functionality will be provided by two buttons
on the transition editting window controlled by the TransitionWindow
and TWButtonListener classes. |
Template created by G. Walton (GWalton@mail.ucf.edu)
on October 8, 1999 and last modified on August 15, 2000
This page last modified by Michael Wales ( Mag7Rule@aol.com ) on November 20, 2000